Programing an app for android that needs user to select date and time or just view a calendar? Android Calendar Library can be of a great help to provide you a widget that you can implement in your app and provide a beautiful widget for your user!
Need some career advice or prepping for an Android developer interview? Hit me up on Topmate.io, and let's chat!
Here is a curated list of 21 such top Android Calendar library with implementation code:
Cosmo Calendar
Cosmo calendar is an awesome library that provides user the ability to select a particular date, range or some days
To add this library in your project, add the following line of code to your build.gradle file:
dependencies {
implementation 'com.github.applikeysolutions:cosmocalendar:1.0.4'
}
Click here to see sample project using Cosmo Calendar library.
Time Range Picker
DateTime Picker tries to offer you the date and time pickers as shown in IOS, with an easy themable API.
Support for Android 4.1 and up. (Android 4.0 was supported until 3.6.4)
Event Calendar
This is the simple library of calender, where you can set event on specific date. And this calender you can customize all section.
By below options you can change or customize the view:
Attributes | Purpose |
---|---|
app:selector_color | To change Selector color |
app:current_month_day_color | To change current month days color |
app:off_month_day_colorr | To change off month days color |
app:week_name_color | To change week name color (sun,mon etc) |
app:month_colorn | To change month title color |
app:next_icon | To change next month icon |
app:previous_icon | To change previous month icon |
app:app:calender_background | To change total calender background color |
app:selected_day_text_color | To selected day text color |
Add the following to build.gradle app file:
dependencies {
implementation 'com.github.mahimrocky:EventCalender:v1.0.0'
}
Also add this code to your Root Gradle file:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Kizitonwose CalenderView
Kizitonswose CalenderView is a highly customizable calendar library for Android, which is built on top of the RecyclerView Widget.

Some features of this library are as follows:
- Single or range selection – The library provides the calendar logic which enables you to implement the view whichever way you like.
- Week or month mode – show 1 row of weekdays, or any number of rows from 1 to 6.
- Disable desired dates – Prevent selection of some dates by disabling them.
- Boundary dates – limit the calendar date range.
- Custom date view – make your day cells look however you want, with any functionality you want.
- Custom calendar view – make your calendar look however you want, with whatever functionality you want.
- Custom first day of the week – Use any day as the first day of the week.
- Horizontal or vertical scrolling mode.
- Month headers and footers – Add headers/footers of any kind on each month.
- Easily scroll to any date or month view using the date.
- Use all RecyclerView customisations (decorators etc) since CalendarView extends from RecyclerView.
- Design your calendar however you want. The library provides the logic, you provide the views.
To get started with this library, add the following lines of code to your build.gradle file:
android {
defaultConfig {
// Required ONLY when setting minSdkVersion to 20 or lower
multiDexEnabled true
}
compileOptions {
// Flag to enable support for the new language APIs
coreLibraryDesugaringEnabled true
// Sets Java compatibility to Java 8
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
coreLibraryDesugaring 'com.android.tools:desugar_jdk_libs:<latest-version>'
implementation 'com.github.kizitonwose:CalendarView:<latest-version>'
}
Also, add the JitPack repository to your project-level build.gradle:
allprojects {
repositories {
google()
jcenter()
maven { url "https://jitpack.io" }
}
}
Next add the following XML Code to the layout file where you wish to display the Calender:
<com.kizitonwose.calendarview.CalendarView
android:id="@+id/calendarView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cv_dayViewResource="@layout/calendar_day_layout" />
Also, you can create your own day View in the layout resource file just like any recyclerview viewHolder:
<TextView
android:id="@+id/calendarDayText"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="16sp"
tools:text="22" />
You can find more details about the library here: Kizitonwose CalenderView Library
BottomSheetPickers
BottomSheetPickers is a tiny Android library for adding Date and Time Pickers as Modal BottomSheetDialogs with From and To ranges.
The library is inspired by Titto Jose’s TimeRangePicker.
Customisation options available with this app are as follows:
BottomSheetTimeRangePicker
.tabLabels(startTabLabel = "Hello", endTabLabel = "World")
.doneButtonLabel("Ok")
.startTimeInitialHour(2)
.startTimeInitialMinute(11)
.endTimeInitialHour(10)
.endTimeInitialMinute(22)
.newInstance(this, DateFormat.is24HourFormat(this))
.show(supportFragmentManager, tagBottomSheetTimeRangePicker)
To implement this library in your project, add these line of code to your build.gradle file:
dependencies {
implementation 'com.github.adawoud:BottomSheetTimeRangePicker:latest-release'
}
SlyCalendarView
A calendar that allows you to select both a single date and a period. Calendar allows you to change colors programmatically without reference to the theme.
To implement this library in your project, add to your build.gradle file:
dependencies {
implementation 'com.github.psinetron:slycalendarview:${version}'
}
Add the JitPack repository to your build file
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Sleep Schedule Picker
Making an alarm app, this android calendar library can help!
To implement this library in your project, add this to your build.gradle file:
dependencies {
implementation 'com.sleepsci:timepicker:1.0.2'
}
WeekDaySelector
This android calendar library help to pick date of per week base date.
To implement this library in your project, add these line of codes to your build.gradle file:
dependencies {
implementation 'com.github.mahimrocky:WeekDaySelector:1.0.0'
}
SwipePicker (Kotlin)
It’s a widget for Android that allows the user to enter different values, such as: time, date, number, without additional dialog windows using the swipe gestures.
To use SwipePicker in your projects, simply add the library as a dependency to your module build.gradle.
dependencies {
implementation 'one.xcorp.widget:swipe-picker:1.1.0'
}
Horizontal Calendar View (Kotlin)
Horizontal Calender View is a library for android.
Add following Block in root in build.gradle(Module:app)
dependencies {
implementation 'com.github.mybringback22:HorizontalCalendarView-Android-:0.1.0'
}
LazyDatePicker (Java)
This is an Android project to offer an alternative to the native Android Date Picker.
To make a lazy date picker add LazyDatePicker in your layout XML and add LazyDatePicker library in your project or you can also grab it via Gradle:
dependencies {
implementation 'com.mikhaellopez:lazydatepicker:1.0.0'
}
CalendarDateRangePicker
An Android Library to pick dates range, that helps user to select range from future dates.
Features:
- Date selection
- Swipe to change month
- Full customization
- Small in size
- Material design support
- Resolution support
The library has following XML attributes
Attribute | Type | Desciption |
---|---|---|
title_color | Color | Title color |
week_color | Color | Week text color |
range_color | Color | Date range color |
selected_date_circle_color | Color | Selected date text circle color |
selected_date_color | Color | Selected date text color |
default_date_color | Color | Default date text color |
range_date_color | Color | Date text color when it falls into range |
disable_date_color | Color | Disable date color |
enable_time_selection | Boolean | true to enable time selection else false |
text_size_title | Dimension | Title size |
text_size_week | Dimension | Week text size |
text_size_date | Dimension | Date text size |
header_bg | Drawable | Header background |
week_offset | Dimension | To set week start day offset |
enable_past_date | Boolean | Enable/Disable past date’s by default its value is false |
editable | Boolean | When true user can edit. By default its value is true |
Add following line to App level gradle:
dependencies {
compile 'com.archit.calendar:awesome-calendar:1.1.4'
}
Collapsible-Calendar-View-Android
Add it in your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Add the dependency in your app build.gradle
dependencies {
implementation 'com.github.shrikanth7698:Collapsible-Calendar-View-Android:v1.0.0'
}
SectionCalendarView
Custom CalendarView with selecting StartDay, EndDay for Android Application, written in Java and Kotlin
rootProject/build.gradle
allprojects {
repositories {
maven { url 'https://jitpack.io' }
}
}
app/build.gradle
dependencies {
implementation 'com.github.WindSekirun:SectionCalendarView:1.0.5.1'
}
ScrollHmsPicker
A simple HMS time picker with scrolling.
Add it in your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Step 2. Add the dependency =>
dependencies {
implementation 'com.github.DeweyReed:ScrollHmsPicker:1.0.3'
}
Calendar Picker
This Android Calendar Library can preset a selected day. Can customize almost all text size, color, bg color, and month title.
Update:
v1.1: If no preset day, hide Selected button. Show it when a day be clicked.
v1.1.2: Remove no needed example code and intent filter which create no needed launch icon.
Add it in your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Step 2. Add the dependency =>
dependencies {
implementation 'com.github.maxyou:CalendarPicker:v1.1.2'
}
Linear Time Picker Library (Java)
Gorgeous Time and Date picker library inspired by the Timely app
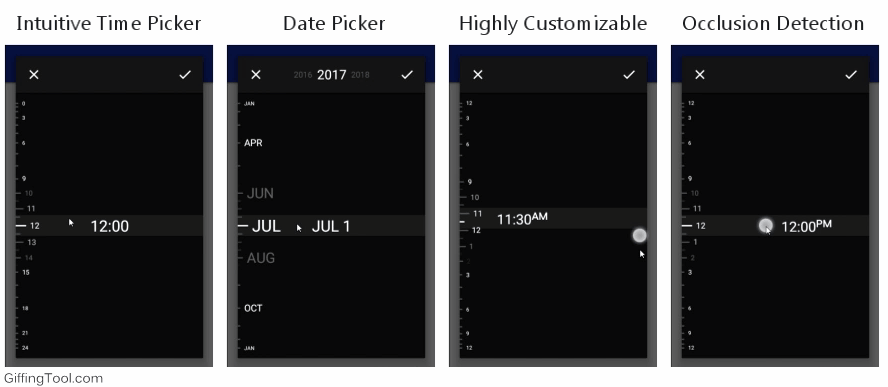
The library comes with following customization:
// Set the background color of the dialog (1)
setDialogBackgroundColor(int color)
// Set the background color of the picker inside the dialog (2)
setPickerBackgroundColor(int color)
// Set the color of the unselected lines in the linear dial (3)
setLineColor(int color)
// Set the color of all the displayed text
setTextColor(int color)
// Show a short 10 second automated tutorial to onboard the user
setShowTutorial(boolean showTutorial)
// Set the background color of the "handle" (4)
setTextBackgroundColor(int color)
// Set the color of the two buttons at the top of the dialog (5)
setButtonColor(int color)
// Register a callback when the selection process is completed or canceled
setButtonCallback(new LinearTimePickerDialog.ButtonCallback() {
@Override
public void onPositive(DialogInterface dialog, int hour, int minutes) {
Toast.makeText(MainActivity.this, "" + hour + ":" + minutes, Toast.LENGTH_SHORT).show();
}
@Override
public void onNegative(DialogInterface dialog) {
}
})
Add it in your root build.gradle at the end of repositories:
dependencies {
compile 'net.codecrafted:lineartimepicker:1.0.0'
}
DatePicker Timeline
First, add jitpack in your build.gradle at the end of repositories:
repositories {
// ...
maven { url "https://jitpack.io" }
}
Then, add the library dependency:
dependencies {
compile 'com.github.badoualy:datepicker-timeline:c6dcd05737'
}
CustomizableCalendar
This library allows you to create a completely customizable calendar. You can use CustomizableCalendar to create your calendar, customizing UI and behaviour.
Features
- Custom header (should be implemented by the user);
- Different sub view (month name by default);
- Add new weekly days view;
- Ability to add new date view;
- Possibility to implement selection on day click;
- Possibility to implement weekly day calculation;
- Receive updates of Calendar (with AUCalendar);
- Every change to AUCalendar is notified and automatically refreshes UI;
First, add jitpack in your build.gradle at the end of repositories:
repositories {
// ...
maven { url "https://jitpack.io" }
}
Then, add the library dependency:
dependencies {
compile 'com.github.MOLO17:CustomizableCalendar:v0.1.4'
}
NumberPadTimePicker
Make time selections in Android by typing them.
As you type or remove digits, number keys and the “OK” button are enabled or disabled to prevent you from setting invalid times. The time separator (e.g. “:”) character is dynamically formatted into the correct position.
Available as an alert dialog, a bottom sheet dialog, and as a plain View.
First, add jitpack in your build.gradle at the end of repositories:
repositories {
// ...
maven { url "https://jitpack.io" }
}
Then, add the library dependency:
dependencies {
compile 'com.github.philliphsu:numberpadtimepicker:1.1.1'
}
MeowDateTimePicker
This library offers a hijri/shamsi (Iran Calendar) Date Picker and a normal time picker designed on Google’s Material Design Principals For Pickers for Android 4.0.3 (API 15) + and forked from mohamad-amin/PersianMaterialDateTimePicker
The best part about this library is that it supports custom fonts!
build.gradle (project path)
buildscript {
repositories {
jcenter() // this line need
}
....
}
Android Studio 3+
dependencies {
implementation 'ir.he.meowdatetimepicker:library:1.0.2'
}
Also don’t forget to add permission in AndroidManifest.xml file to vibrate.:
<manifest >
<uses-permission android:name="android.permission.VIBRATE" />
<application
android:name=".MyApplication"
...
</application>
</manifest>
Now go do some awesome stuff!
Interested in creating your own Custom Android Calendar, see here: How to Create Custom Calendar in Android Example
1 comment